Containers in Flutter
In this tutorial, we are going to explore the usage of containers in Flutter.
We assume you have installed Flutter and are using VS Code, but if you have not done so, you can follow this simple tutorial to install Flutter and setup VS Code.
https://flutter.dev/docs/development/tools/vs-code
The skeletal code for this series can be found in my Github repo as well. You can clone the repo to follow along this tutorial. If you have no experience in cloning a repo or working with Github, checkout my tutorial here.
https://github.com/Prakashash18/flutterBlogs/tree/main/flutter_containers
Containers in Flutter are in some ways similar to div tags in HTML (for those who come from a web dev background). They are customisable UI widgets.
The build method’s body below shows a Center Widget with a Container as the child. The Container has a Text Widget as a child with the message as “Hey there”.
@override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( //body child: Container( child: Text("Hey There"), )), // This trailing comma makes auto-formatting nicer for build methods. ); }
Executing the code above will produce the output shown below.
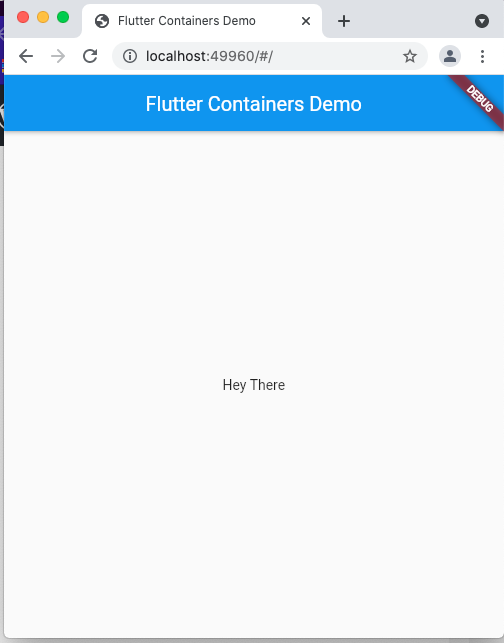
Containers in Flutter – Color
Containers will wrap around the child if the height and width are NOT specified. You can see this by assigning a color to the container.
Center( child: Container( color: Colors.blue, //blue color is assigned child: Text("Hey There"), )),
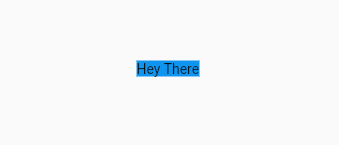
As shown, the Text Widget is wrapped with a color of blue.
Containers in Flutter – Fixed Width and Height
Now in order to spread out the container, a width and height can be assigned to the container and in the code snippet, you can assign a fixed width and height of 100 pixels to see a box.
Center( child: Container( width: 100, height: 100, color: Colors.blue, child: Text("Hey There"), )),
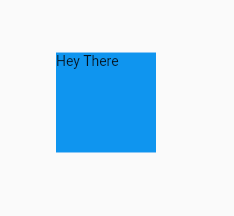
Containers in Flutter – Text Centralisation
The text as shown is positioned at the top left of the container. To position it to the center of the container, wrap the text widget with a center widget as shown.
Center( child: Container( width: 100, height: 100, color: Colors.blue, child: Center(child: Text("Hey There",)), )),
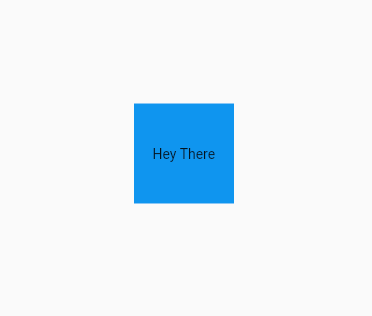
Container- Borders
The BoxDecoration class comes in handy when decorating a container.
The container’s decoration property accepts a BoxDecoration class. It’s important to note that the Container color property and the decoration property cannot be specified at the same time; thus the BoxDecoration class will be used to specify the color as shown.
Center( child: Container( width: 100, height: 100, decoration: BoxDecoration( color: Colors.blue, //color is part of BoxDecoration border: Border.all( width: 10, color: Colors.red, )), child: Center( child: Text( "Hey There", )), )),
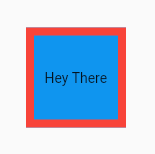
Container Border Radius
The borders can also be curved by introducing a borderRadius property in the BoxDecoration class.
Center( child: Container( width: 100, height: 100, decoration: BoxDecoration( color: Colors.blue, borderRadius: BorderRadius.circular(5), //border radius border: Border.all( width: 10, color: Colors.red, )), child: Center( child: Text( "Hey There", )), )),
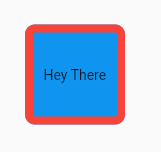
We can also specify which sides we want the radius to appear by using the method only of the BorderRadius class. As such, in the code snippet below, only the topRight and topLeft have a radius of width 5.
Center( child: Container( width: 100, height: 100, decoration: BoxDecoration( color: Colors.blue, borderRadius: BorderRadius.only( topLeft: Radius.circular(5), topRight: Radius.circular(5)), //using the only method border: Border.all( width: 10, color: Colors.red, )), child: Center( child: Text( "Hey There", )), )),
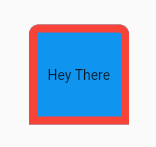